A Walkthrough to Go Syntax and Features
A brief history of the Go programming language, which was founded by the legends of computer science: Ken Thompson, Rob Pike, and, lastly, Robert Griesemer. Go development was started in 2007 and was funded by Google even until now.
Go is an open source, general purpose programming language that has Google’s support. Being open source does not automatically imply that it is open to collaboration. The Go team is in charge of the development’s overall direction. Before implementing or making critical changes to the language’s features and tooling, I assume they listen to suggestions from the Go Community. Currently, Russ Cox, a Google tech lead, is in charge of the development.
What is the Programming Paradigm of Go?
Go is a multi-paradigm language, but most of its concepts are in the procedural paradigm. Though it has object-oriented programming concepts, the Go team chose composition over inheritance, and it doesn’t have a class
data type.
A Friendly Reminder
Before starting with the tedious learning process, allow me to tell you that if you are learning this programming language to land an entry level job in the Philippines, then I suggest that you pick a more mature and popular language such as Java, C++, PHP, Javascript, and Python.
Preparing the Development Environment
Let’s start by installing the Go compiler and toolchains. There are several installation procedures for Go, depending on the operating system you’re using. No need to worry, it’s pretty simple and intuitive, but I doubt it’ll be as simple as installing Java or Python. Let’s get started!
Installation Procedure
These are the go compiler installation procedures, choose the procedure that is appropriate for the operating system you are currently using or it will not work.
But before all of that, download a binary release that is suitable for your system. Visit https://go.dev/dl/
to download it.
Note: The file extension for debian based is .deb
, for fedora and rhel is .rpm
and for windows is .msi
Windows
- Run the file with
.msi
extension that you have downloaded. - Follow through the prompts to install go.
- The installation directory will be in
Program Files
orProgram Files (x86)
. - Verify if the installation was successful by running
go version
in cmd.
Linux
- Use
tar
command to decompress the file and add-C
flag to copy the decompressed file to a specific directory.
tar -C /usr/local -xzf go1.20.linux-amd64.tar.gz
- Add a new line to your environment variables.
export PATH=$PATH:/usr/local/go/bin
- Open terminal and run
go version
. To verify the installation. - You are ready to
GO
!
Hmmm… What else do you need?
Yes! A code editor!
Just pick whatever code editor you prefer.
Visual Studio Code
I highly recommend Microsoft’s code editor, Visual Studio Code. Because of its plugin-based extensibility. It’s made with web technologies like html, CSS, and javascript, as well as electronjs. In terms of performance, this code editor can compete with any modern code editor. BTW theming is my favorite feature, yeaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa THEMES! Who doesn’t enjoy a good theme? If you don’t like themes, you’re insane. Make an appointment with a psychiatrist in your area (just kidding). If you don’t have a code editor installed, use this one instead.
Lapce
A promising new code editor written in Rust that is extremely fast. Though I don’t think it looks good right now. Perhaps the team should modernize its UI design a little more. This code editor is definitely worth a try if you want to try something new.
NeoVim
If you prefer a modal code editor and code faster without a GUI, this code editor is for you. Personally, I use it to edit the configuration files of my installed Linux distro. It’s very useful for me in terms of modifying configuration files, and it’s much faster than opening a GUI-based editor. I use Linux and prefer to run code editors from the terminal, so neovim is very convenient for me.
Notepad++
I don’t use it much because I don’t use Windows. However, it is quite lightweight, and if you have an old computer, this code editor will run as smoothly as butter.
If you’ve already chosen your weapon… er, code editor. Let’s start with the basics of Go.
The Basics!
Ohhh exciting right?.. NO?
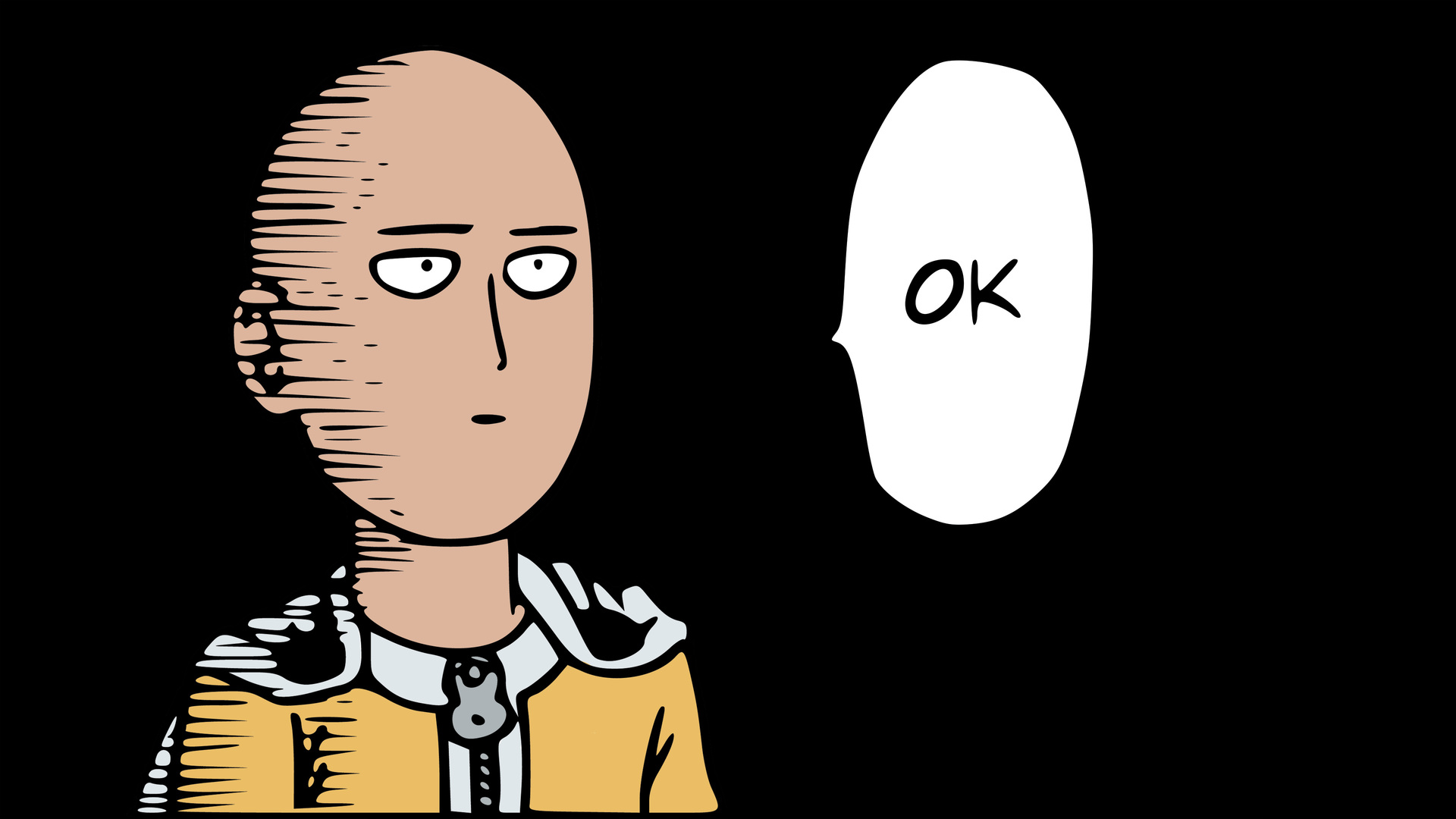
Note: I’m using comments to annotate the source code. If the line starts with //
you are reading a comment. The compiler ignores lines that begin with a comment symbol.
Data Types
To define a type in Go during variable declaration, we have to write it right after the identifier. For example:
var name string // the string is our defined type for the variable indentied as "name"
var age int
var truth bool
We can also define multiple variables of the same type in a single line. The correct way to do that is to seperate every identifiers with commas.
var name, address, gender string
1. String type:
String type is an array of characters. A low level language like C does not support string types by default. Go stores string as immutable slice of bytes.
- the keyword is: string
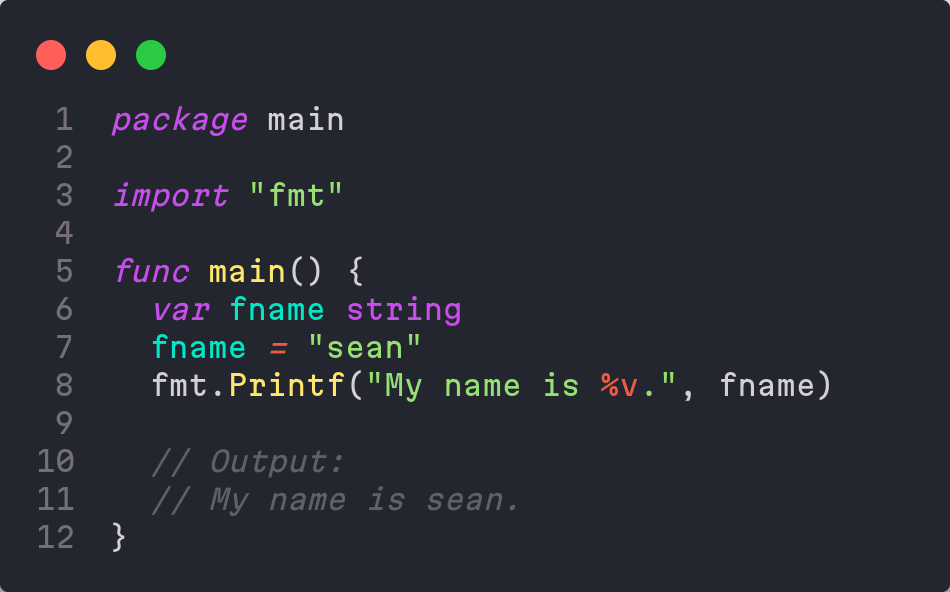
2. Boolean type:
A Boolean type’s value can be true
or false
. It can be use as a return value from a comparison or relational expression.
- the keyword is: keyword: bool
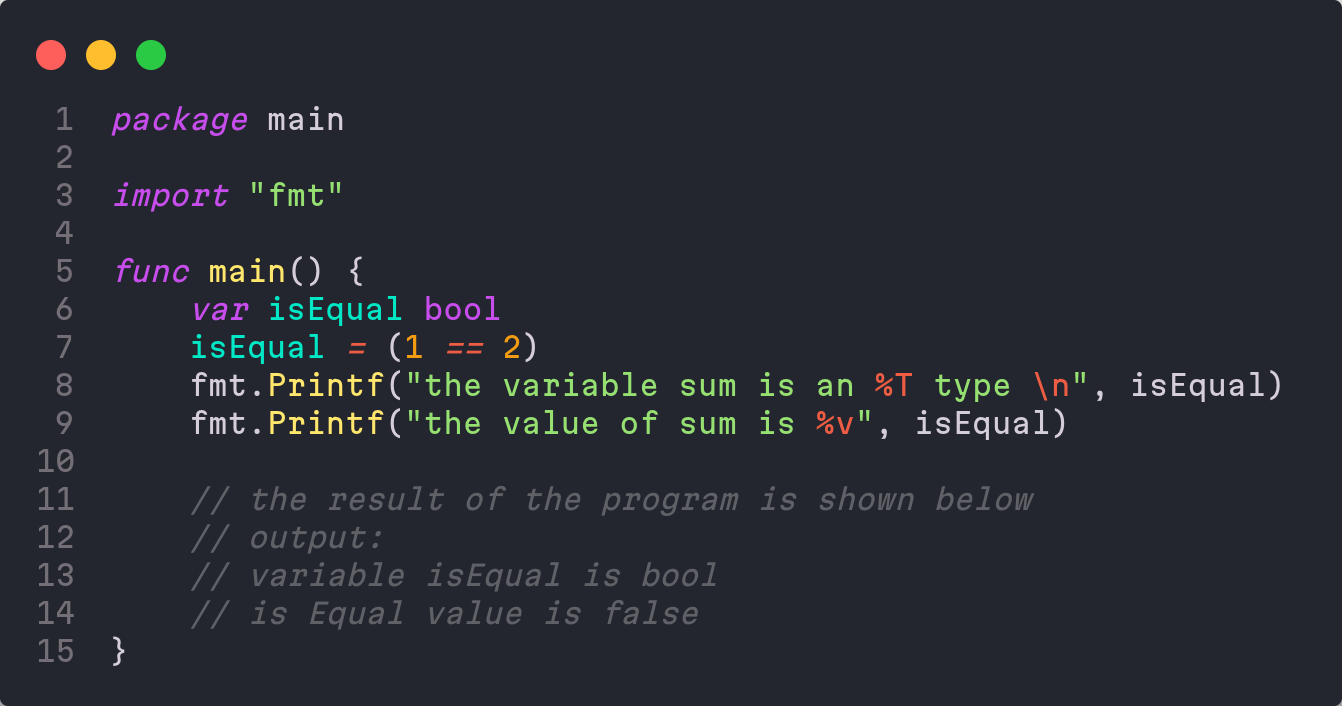
3. Integer numeric types:
An integer is a set of numbers including all the positive and negative numbers. We can do arithmetic operations with integers.
- the keywords are: int, uint, int8, uint8, int16, uint16, int32, uint32, int64, uint64, and uintptr.
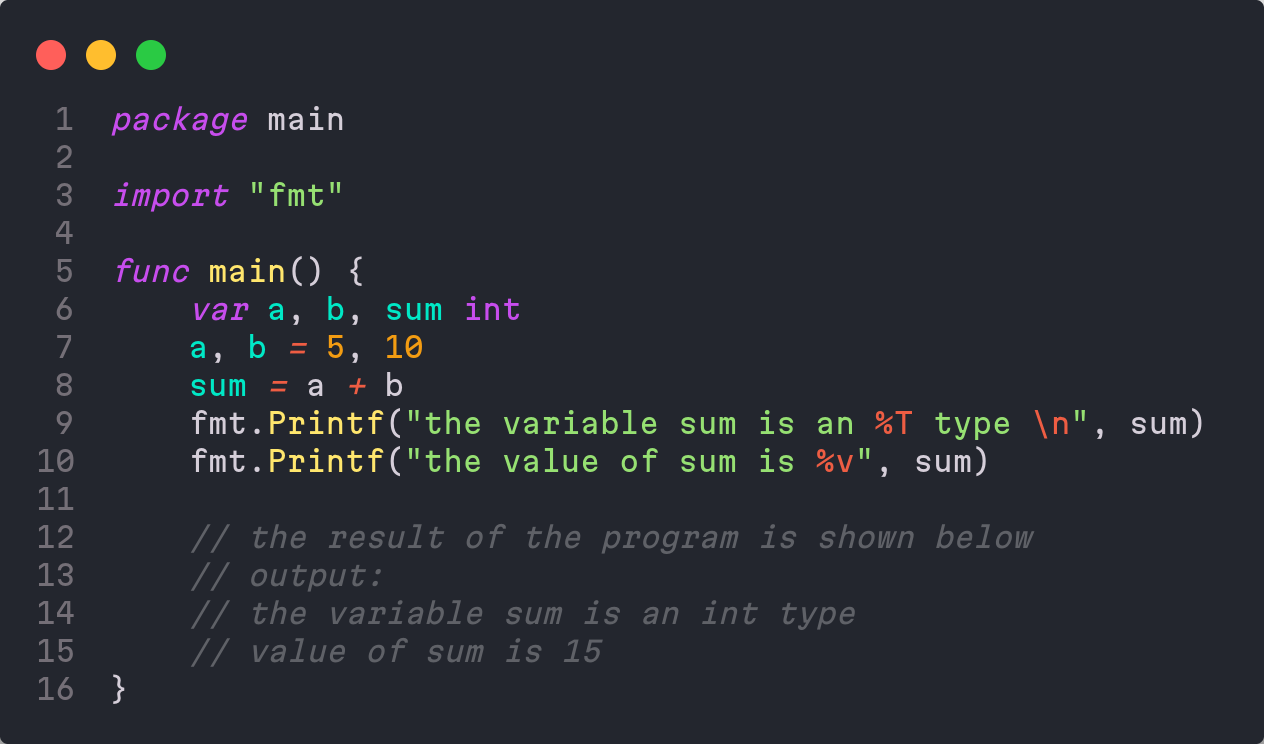
4. Floating point types:
Floating point are numeric type that is either positive or negative number and has decimal points. We can also perform arithmetic operations with floating points, which is similar to integers.
- the keywords are: float32 and float64.
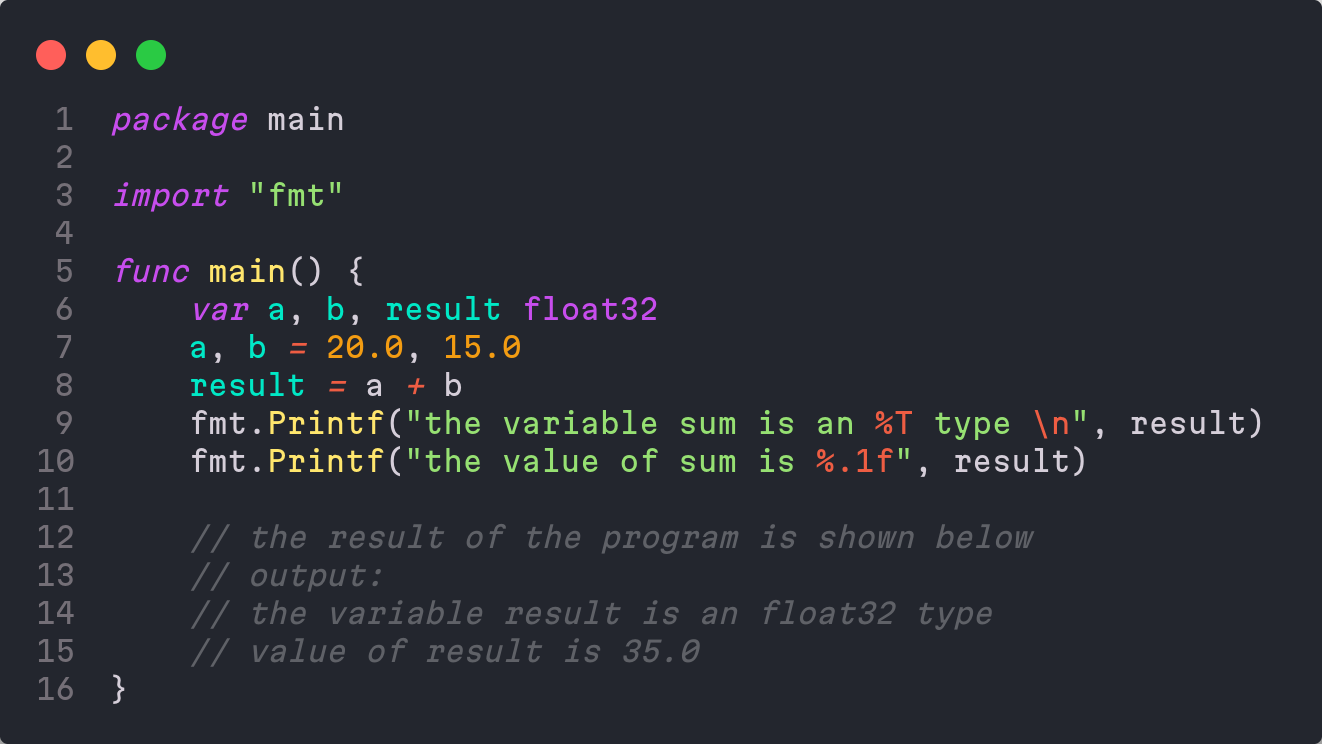
5. Complex numeric types:
Complex numeric types are created from given real and imaginary parts.
- the types are: complex64 and complex128
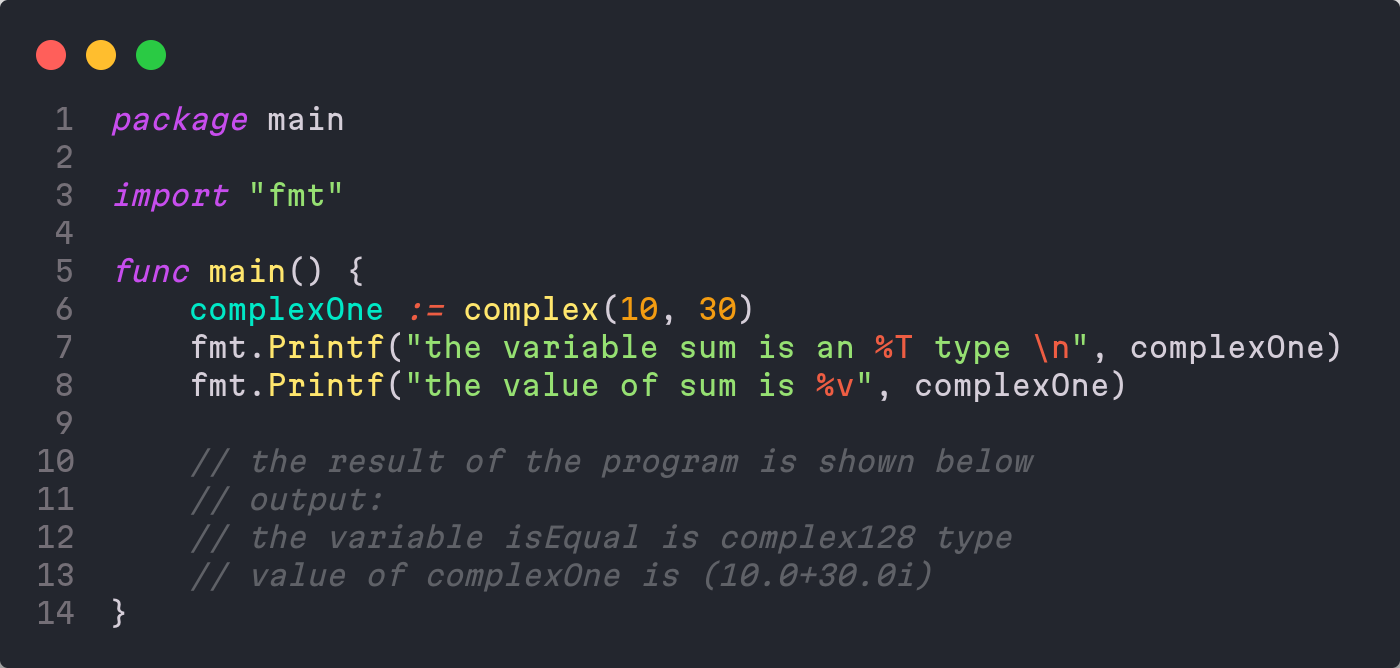
Arithmetic Operators
The compiler reads your line of code from left to right and from top to bottom. You always have to follow that rule when you write code in order to avoid syntax errors.
Operator Precedence
This is a very important rule before we can start doing arithmetic expressions.
These are the order of operations:
- Parentheses
- Exponents
- Multiplication / Division
- Addition / Subtraction
We can use the mnemonic called PEMDAS
to easily remember the order of operations.
Arithmetic Expressions
1. Addition
result = 10 + 10
fmt.Println(result)
// 20
2. Subtraction
result = 10 - 5
fmt.Println(result)
// 5
3. Multiplication
result = 5 * 20
fmt.Println(result)
// 100
4. Division
result = 10 / 5
fmt.Println(result)
// 2
Mutable, Immutable Variable Declaration and Type Inference
In Go, variables are mutable by default and the value that has been initialized can be changed later. In order to declare a scalar value (immutable) we are going to use the const
keyword.
Mutable variable declaration
It uses the var
keyword for declaring a variable.
var name string
Immutable variable declaration
Now, for the immutable.
The keyword used for the immutable variable declaration is const
.
const name = "Sean"
const res = true
And so if we attempt to assign another value to our constants it will not compile because of an error.
name = "Miranda" // This will result to an error.
Type Inference
Type inference is inferring the type of an initialized variable, where you no longer have to define its type. It can be done in two different ways. Check the line of code below.
By using the var
keyword and intializing the value of our variable.
var name = "Sean"
Or by using the so called, walrus operator :=
.
name := "Sean"
Basic I/O Operations
Go provides a package called fmt
for basic I/O operations. These are the 2 most used in output operations from the fmt
package.
In order for us to invoke the Print
statement variations in our code, we’re gonna have to import the fmt
package by using the import
keyword.
1. The first one is fmt.Println()
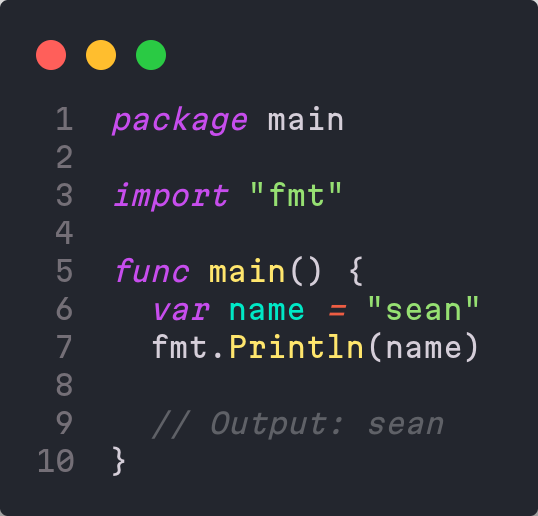
2. Second is fmt.Printf()
.
In the code below, we used the verb %v
for string interpolation. It sucks that go only use this type of string interpolation.
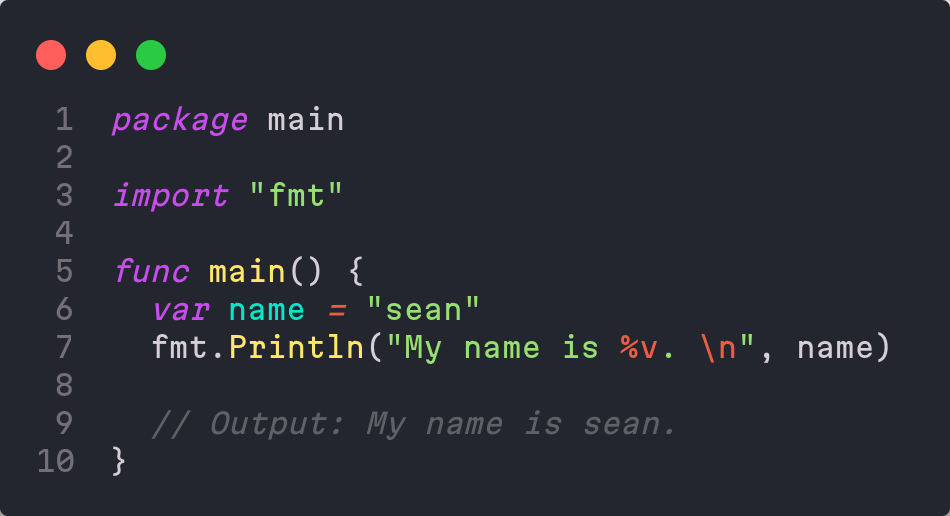
Control Flow
The control flow statements are used to control the flow of your program. So the flow wouldn’t be linear. It passes through condition checks and executes the underlying code blocks when the conditions have been met.
Single if-else statements
Here’s the structure of an if-else statement.
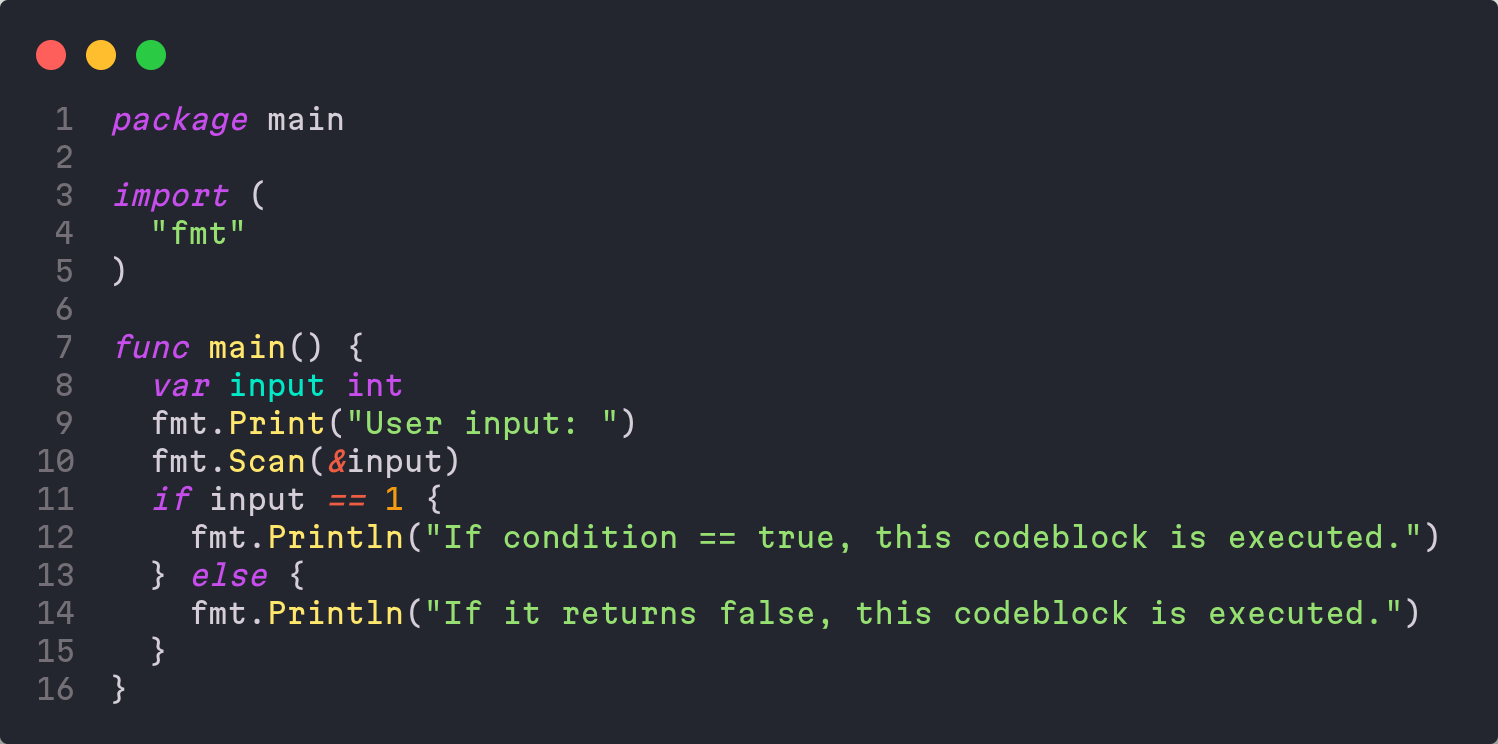
Take a look at line 11, it starts with the if
keyword and then the condition is written beside it. If that condition returns true
then the codeblock under the if
statement is executed. Otherwise, the else
codeblock is executed if it returns false
.
Nested if statements
If you are writing a more complex flow in your code, then its viable to use nested if statements.
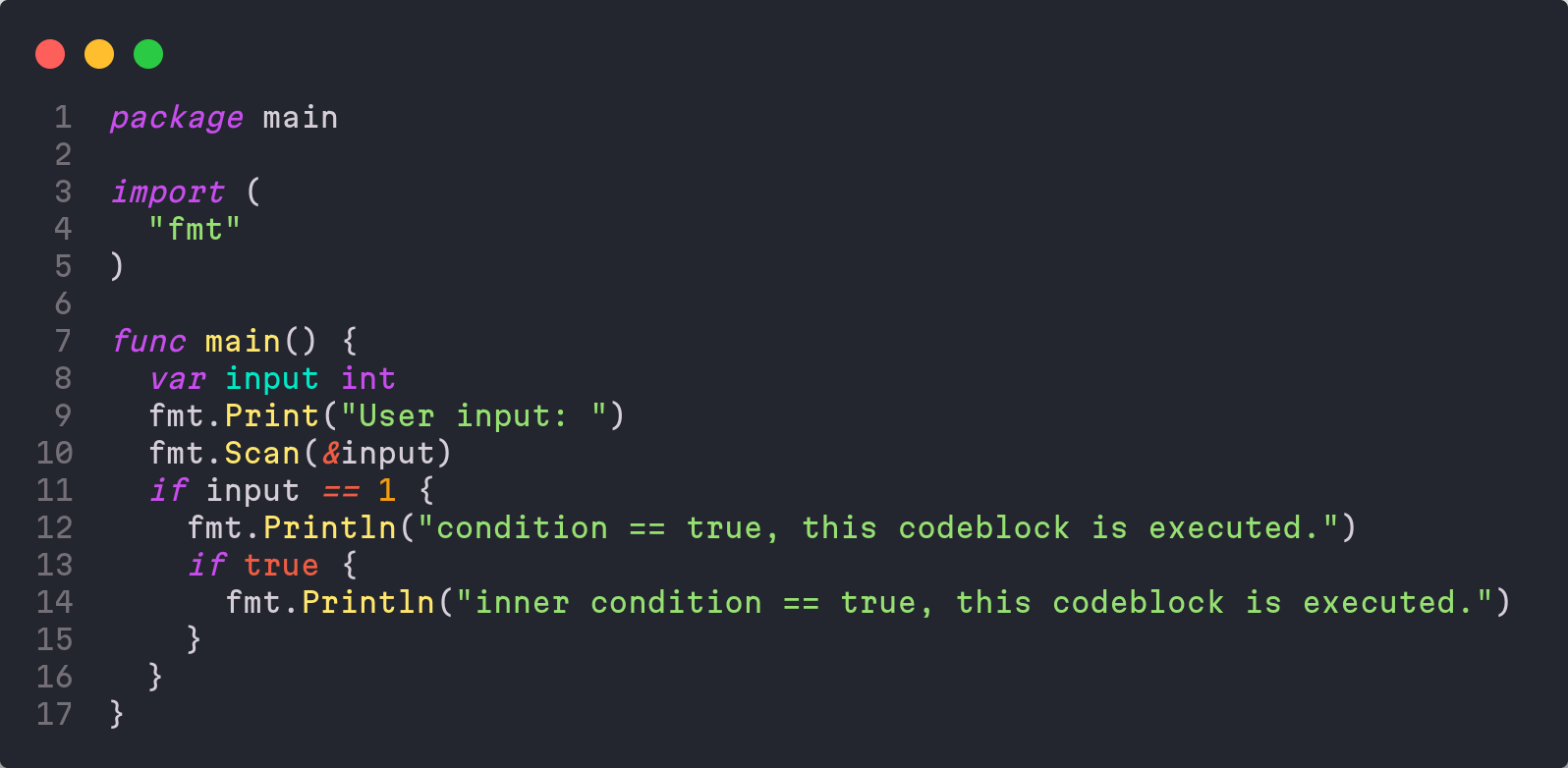
Loops and Iterations
The general purpose for loop statement
Go only supports the for
keyword in all loop/iterations use cases, such as iterating through a linear data structure, e.g., a slice of bytes or an array. The other use cases won’t be covered since this is only an introductory blog.
The code below is iterating through an array of integers with our general purpose for
statement.
In line 8, we declared and initialized our array of integers with a fixed size of 5 elements.
A for
statement is structured with 3 statements (or expressions).
It starts with the initialization of our starting index value, then moves to check the condition, if the condition returns true, then it executes the codeblock once and increments our index value. It passes through it all again until the condition returns false.
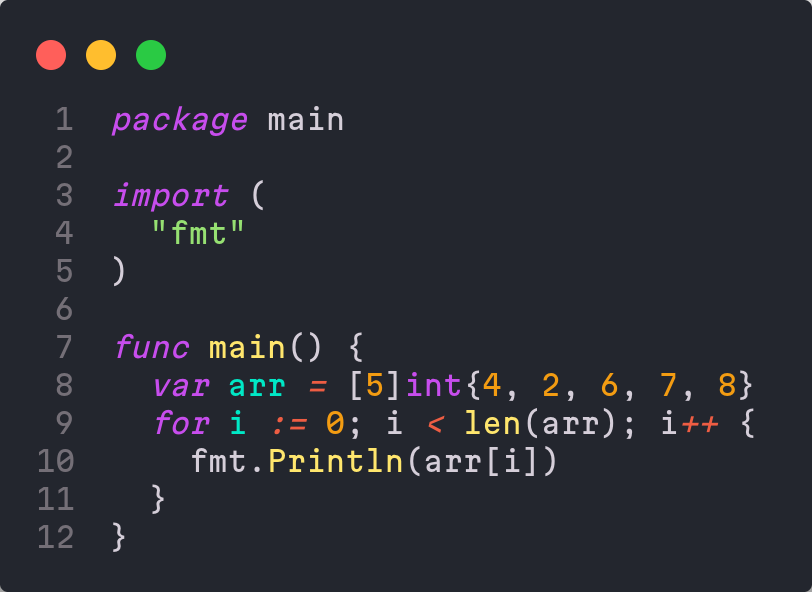
That’s all for now. Please look forward to my next post.
Summary
We covered the most important introductory topics for learning Go programming language. The list of topics covered are listed below.
- Installation: We prepared the development environment for the go programming language, and also provided other procedures suitable to every systems.
- Code editors: Listed few code editors that are very popular and can be used for writing go code.
- Basics: Introduction to the basics of Go, such as data types, I/O operations, Control flow and Iterations.
Next Post
We will be covering data structures and type system overviews in the second part of this blog post. I might also write a different blog post about my opinion and ideas regarding the proposal of telemetry in the Go toolchain.